This chapter excerpt from
ASP.NET Data Presentation Controls Essentials by Joydip Kanjilal,
is printed with permission from Packt Publishing
, Copyright 2007.
Binding Images Dynamically
Let us now see how we can display images using the DataList control. Here is a
situation where this control scores over the other data-bound controls as you
can set the RepeatDirection property of this control to Horizontal so that we
can display the columns of a particular record in one single row.
The following screenshot illustrates how the output of the application would
look when it is executed:
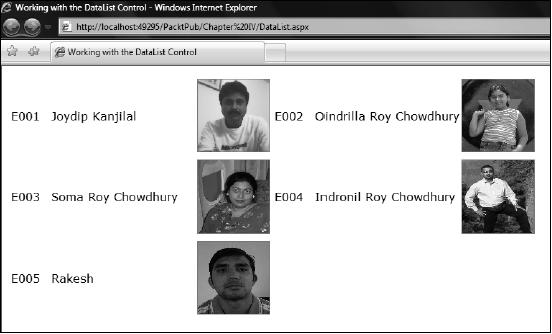
We will now discuss how we can implement this application that displays the
employee details, like code, name, and the individual's photo. We need an Image
control that we will use inside the ItemTemplate of the DataList control in
use. Here is how you can use the Image control.
<img src='<# DataBinder.Eval(Container.DataItem, "EmpName") %>.png'
style="height:100px;width:100px;border:1px solid gray;"/>
Note that all the images have a primary name corresponding to the employee's
name with a .png extension. The complete source code of the DataList control in
your .aspx fi le would be similar to what follows:
<asp:DataList ID="DataList1" runat="server" RepeatColumns="2"
RepeatDirection="Horizontal">
<ItemTemplate>
<table id="Table1" cellpadding="1" cellspacing="1" visible ="true">
<tr>
<td width="50px">
<p align="left">
<asp:Label ID="lblEmpCode" runat ="server" CssClass="LabelStyle" Text='
<%# DataBinder.Eval(Container.DataItem, "EmpCode")%>'>
</p>
<td>
<td width="200px">
<p align="left">
<asp:Label ID="lblEmpName" runat = "server"< BR
>
CssClass="LabelStyle" Text='
<%# DataBinder.Eval(Container.DataItem, "EmpName")%>'>
</asp:Label>
</p>
</td>
<td
width="100px">
<p
align="left">
<img
src= '<%#DataBinder.Eval(Container.DataItem, < BR>
"EmpName") %>.png'
style="height:100px;width:100px;border:1px solid gray;"/>
</td>
</p>
</td>
</table>
</ItemTemplate>
</asp:DataList>
Note the use of the properties RepeatColumns and RepeatDirection in this code
snippet. While the former implies the number of columns that you would like to
display per record in the rendered output, the later implies the direction of
the rendered output, that is, horizontal or vertical.
Binding data to the control is simple. You need to bind the data to this control
in the Page_Load event of this control in your code-behind fi le. Here is how
you do the binding:
DataManager dataManager = new DataManager();
DataList1.DataSource = dataManager.GetEmployees();
DataList1.DataBind();
Wow! When you execute the application, the images are displayed along with the
employee's details. The output is similar to what we have seen in the
screenshot earlier in this section.
In the following sections, we will explore how to Select, Edit, and Delete data
using the DataList control.
Also read
Model View Controller
we will learn about MVC design patterns, and how Microsoft has made our lives
easier by creating the ASP.NET MVC framework for easier adoption of MVC
patterns in our web applications...............
Page Controller Pattern in
ASP.NET
Here it shows how a page controller pattern works in ASP.NET.
MVC Design
MVC, which stands for Model View Controller, is a design pattern that helps us
achieve the decoupling of data access and business logic from the presentation
code , and also gives us the opportunity to unit test the GUI effectively and
neatly, without worrying about GUI changes at all..........
REST: Representation State Transfer
REST means Representational State Transfer, an architectural pattern used to
identify and fetch resources from networked systems such as the World Wide Web
(WWW). The REST architecture was the foundation of World Wide Web. But the term
itself came into being around the year 2000, and is quite a buzzword these
days...........
ASP.NET MVC Framework
The ASP.NET MVC framework was released by Microsoft as an alternative approach
to web forms when creating ASP.NET based web applications...........
Working with the DataList Control
The DataList control like the Repeater control is a template driven, light
weight control, and acts as a container of repeated data items. The templates
in this control are used to define the data that it will contain...............
.NET crystal reports
How do we access crystal reports in .NET?
What are the various components in crystal reports?
What basic steps are needed to display a simple report in crystal?..........
ASP.NET 2.0 Data Controls
One of the important goals of ASP.NET 2.0 is 70% code reduction. The data
controls supplied with ASP.NET 2.0 play an important role in making this
ambitious goal a reality. Data source controls provide a consistent and
extensible method for declaratively accessing data from web pages..............
ASP.NET 2.0 Security Controls
With ASP.NET 2.0, things have changed for the better. For security-related
functionalities, ASP.NET 2.0 introduces a wide range of new
controls..............
|