Book Excerpt: AJAX and the Future of Web Applications
Here we will learn about the following:
|
|
This excerpt from AJAX and
PHP: Building Responsive Web Applications by Filip Chereches-Tosa,
Bogdan Brinzarea, Cristian Darie, Mihai Bucica, is printed with permission from
Packt Publishing, Copyright 2007.
Understanding AJAX
AJAX is an acronym for Asynchronous JavaScript and XML. If you think it doesn't
say much, we agree. Simply put, AJAX can be read "empowered JavaScript",
because it essentially offers a technique for client-side JavaScript to make
background server calls and retrieve additional data as needed, updating
certain portions of the page without causing full page reloads. Figure 1.4
offers a visual representation of what happens when a typical AJAX-enabled web
page is requested by a visitor:
|
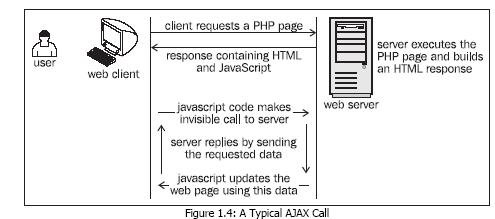
When put in perspective, AJAX is about reaching a better balance between client
functionality and server functionality when executing the action requested by
the user. Up until now, client-side functionality and server-side functionality
were regarded as separate bits of functionality that work one at a time to
respond to user's actions. AJAX comes with the solution to balance the load
between the client and the server by allowing them to communicate in the
background while the user is working on the page.
To explain with a simple example, consider web forms where the user is asked to
write some data (such as name, email address, password, credit card, etc) that
has to be validated before reaching the business tier of your application.
Without AJAX, there were two form validation techniques. The first was to let
the user type all the required data, let him or her submit the page, and
perform the validation on the server. In this scenario the user experiences a
dead time while waiting for the new page to load. The alternative was to do
this verification at the client, but this wasn't always possible (or feasible)
because it implied loading too much data on the client (just think if you
needed to validate that the entered city and the entered country match).
In the AJAX-enabled scenario, the web application can validate the entered data
by making server calls in the background, while the user keeps typing. For
example, after the user selects a country, the web browser calls the server to
load on the fly the list of cities for that country, without interrupting the
user from his or her current activity.
The examples where AJAX can make a difference are endless. To get a better
feeling and understanding of what AJAX can do for you, have a look at these
live and popular examples:
-
Google Suggest helps you with your Google searches. The functionality is pretty
spectacular; check it out at http://www.google.com/webhp?complete=1. Similar
functionality is offered by Yahoo! Instant Search, accessible at
http://instant.search.yahoo.com/.
-
GMail (http://www.gmail.com). GMail is very popular by now and doesn't need any
introduction. Other web-based email services such as Yahoo! Mail and Hotmail
have followed the trend and offer AJAX-based functionality.
-
Google Maps (http://maps.google.com), Yahoo Maps (http://maps.yahoo.com), and
Windows Live Local (http://local.live.com).
-
Other services, such as http://www.writely.com and
http://www.basecamphq.com.
So AJAX is about creating more versatile and interactive web applications by
enabling web pages to make asynchronous calls to the server transparently while
the user is working. AJAX is a tool that web developers can use to create
smarter web applications that behave better than traditional web applications
when interacting with humans.
The technologies AJAX is made of are already implemented in all modern web
browsers, such as Mozilla Firefox, Internet Explorer, or Opera, so the client
doesn't need to install any extra modules to run an AJAX website. AJAX is made
of the following:
-
JavaScript is the essential ingredient of AJAX, allowing you to build the
client-side functionality. In your JavaScript functions you'll make heavy use
of the Document Object Model (DOM) to manipulate parts of the HTML page.
-
The XMLHttpRequest object enables JavaScript to access the server
asynchronously, so that the user can continue working, while functionality is
performed in the background. Accessing the server simply means making a simple
HTTP request for a file or script located on the server. HTTP requests are easy
to make and don't cause any firewall-related problems.
-
A server-side technology is required to handle the requests that come from the
JavaScript client. In this book we'll use PHP to perform the server-side part
of the job.
-
For the client-server communication the parts need a way to pass data and
understand that data. Passing the data is the simple part. The client script
accessing the server (using the XMLHttpRequest object) can send name-value
pairs using GET or POST. It's very simple to read these values with any server
script.
The server script simply sends back the response via HTTP, but unlike a usual
website, the response will be in a format that can be simply parsed by the
JavaScript code on the client. The suggested format is XML, which has the
advantage of being widely supported, and there are many libraries that make it
easy to manipulate XML documents. But you can choose another format if you want
(you can even send plain text), a popular alternative to XML being JavaScript
Object Notation (JSON).
This book assumes you already know the taste of the AJAX ingredients, except
maybe the XMLHttpRequest object, which is less popular. However, to make sure
we're all on the same page, we'll have a look together at how these pieces
work, and how they work together, in Chapter 2 and Chapter 3. Until then, for
the remainder of this chapter we'll focus on the big picture, and we will also
write an AJAX program for the joy of the most impatient readers.
AJAX brings you the following potential benefits when building a
new web application:
-
It makes it possible to create better and more responsive websites and web
applications.
-
Because of its popularity, it encourages the development of patterns that help
developers avoid reinventing the wheel when performing common tasks.
-
It makes use of existing technologies.
-
It makes use of existing developer skills.
-
Features of AJAX integrate perfectly with existing functionality provided by
web browsers (say, re-dimensioning the page, page navigation, etc).
Common scenarios where AJAX can be successfully used are:
-
Enabling immediate server-side form validation, very useful in circumstances
when it's unfeasible to transfer to the client all the data required to do the
validation when the page initially loads.
-
Creating simple online chat solutions that don't require external libraries
such as the Java Runtime Machine or Flash.
-
Building Google Suggest-like functionality
-
More effectively using the power of other existing technologies.
-
Coding responsive data grids that update the server-side database on the fly.
-
Building applications that need real-time updates from various external
sources.
Potential problems with AJAX are:
-
Because the page address doesn't change while working, you can't easily
bookmark AJAX-enabled pages. In the case of AJAX applications, bookmarking has
different meanings depending on your specific application, usually meaning that
you need to save state somehow (think about how this happens with desktop
applications— there's no bookmarking there).
-
Search engines may not be able to index all portions of your AJAX application
site.
-
The Back button in browsers, doesn't produce the same result as with classic
web applications, because all actions happen inside the same page.
-
JavaScript can be disabled at the client side, which makes the AJAX application
nonfunctional, so it's good to have another plan in your site, whenever
possible, to avoid losing visitors.
More Related Links
Explain
ASP.NET Ajax Framework.
Answer - ASP.NET Ajax Framework is used for implementing the
Ajax functionality......
Explain
limitations of Ajax.
Answer - Back functionality cannot work because the dynamic
pages don’t register themselves to the browsers history engine..........
What
is the role of Script Manager in Ajax?
Answer - Script Manager, as the name suggests is used to manage
the client side script of Ajax.............
Understanding AJAX
AJAX is an acronym for Asynchronous JavaScript and XML. If you think it doesn't
say much, we agree. Simply put, AJAX can be read "empowered JavaScript",
because it essentially offers a technique for client-side JavaScript to make
background server calls and retrieve additional data as needed, updating
certain portions of the page without causing full page reloads.............
Rich user interfaces and AJAX
Rich user interfaces can be achieved by using a combination of dynamic HTML
elements such as HTML and JavaScript. However, the scope of such an interface
is limited to client-side behavior and has minimal functional implications due
to the lack of server-side interactions.
AJAX in JBoss portal
AJAX has gained tremendous popularity in the traditional web application
development world due to the richness and agility that it brings to user
interfaces. Portals, such as JBoss portal, can also gain signifi cantly from
AJAX, in terms of implementation of both behavior and functionality.
|