JSR-286 and AJAX
Among the set of features that the new portlet specifi cation JSR-286 introduces
to make things easier for AJAX implementations, are the options to directly
communicate with the portlet through shared render parameters and resource
serving.
Referring back to the workaround option in JSR-168, the new specifi cation
provides a standard for setting and receiving render parameters, and we no
longer have to deal with manual copying OF context and parameters.
To set the render parameter directly to the response, all we have to do is this:
public class AssetPortlet extends GenericPortlet {
public void processAction(ActionRequest request,
ActionResponse response) {
response.setRenderParameter("rate", "4.5");
}
...
}
To receive render parameters in the portlet directly from the view, we can say:
public class AssetPortlet extends GenericPortlet {
public void render(RenderRequest request,
RenderResponse response)
{
String
rate = request.getParameter("rate");
...
}
...
}
Similarly, the resource servicing option allows us to directly invoke the
portlet from the portlet user interface, as follows:
ResourceURL resourceURL = renderResponse.createResourceURL()
<formaction="<%= resourceURL %> method="POST">
...
</form>
Using ResourceURL invokes a new life cycle method called serveResource()on the
server, as follows:
public void serveResource(ResourceRequest req, ResourceResponse resp)
throws PortletException, IOException
{
resp.setContentType("text/html");
PrintWriter writer = resp.getWriter();
writer.print("<p><b>Response Markup</b>");
writer.close();
}
So, now when we use ResourceURL instead of ActionURL, the implementation of the
asynchronous requests appears as follows:
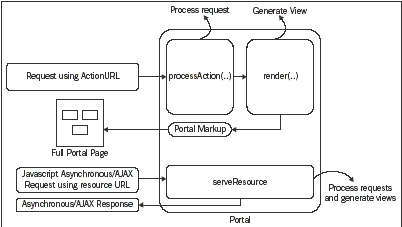
Hence, we can conclude that the following approaches are available for
implementing a portlet user interface:
-
For a traditional, JSR-168 based implementation, ActionURL and RenderURL are
still options, although very limited ones, for asynchronous call
implementations
-
For an AJAX implementation and asynchronous request-response, the ResourceURL
option offered by JSR-286, is the best.
JBoss 2.7.0 offers full support for the JSR-286 Portlet 2.0 specifi cation,
allowing us to implement robust AJAX-based applications without any
workarounds.
With this background, let's dive right into developing a portlet for our
"MyCompany" portal page using AJAX support. In subsequent sections, we will
discuss the confi guration and other in-built AJAX support provided by the
JBoss portal server.
Also read
What is a Portlet? Explain its capabilities.
Explain Portal architecture.
What is PortletSession interface?
What is PortletContext interface?
Why portals?...................
|